Features in the Test Application¶
Preloading the .NET Libraries¶
A slight delay may be experienced the first time the reporting process is launched if the .NET libraries are not currently loaded. These libraries are required by Crystal Reports. Once the libraries are loaded no performance slowdown should be observed. The procedure Activating is augmented in CrystalTest.src so it opens a blank report. This forces preload of the .NET libraries and moves the startup time to the application startup. The splash screen may display a bit longer but the reporting process is significantly faster. You can create a blank report and then copy this code to your application to improve the reporting process.
Procedure Activating
Handle hoF2C
Boolean bCrystalOK bOK
Forward Send Activating
// Verify Flex2Crystal is properly licensed and installed
Get CheckCrystalEnvironment of oCheckForFlex2Crystal to bCrystalOK
If (bCrystalOK) Begin
Get Create U_cFlex2Crystal to hoF2C
Set psReportName of hoF2C to "..\Data\Blank.rpt"
Get OpenReport of hoF2C to bOK
If (bOK) Send CloseReport of hoF2C
Send Destroy of hoF2C
End
End_Procedure
Checking for the Crystal Environment¶
CheckCrystalEnvironment verifies Flex2Crystal is properly licensed and installed, keeps an application from crashing due to an undefined Flex2Crystal condition, and allows you to gracefully detect these conditions and display a dialog box listing the error (for example, “The trial license has expired.”).
An example of including this call and displaying a dialog box if an error occurs is shown in the OnClick procedure. Without this check numerous DataFlex errors may occur and their cause may be difficult to track down.
Procedure OnClick
Boolean bReportOK bCrystalOK
String sReportName
Get CheckCrystalEnvironment of oCheckForFlex2Crystal to bCrystalOK
If (bCrystalOK) Begin
Set psReportName of oCrystalReport to "..\Data\ Blank.rpt"
Send RunReport of oCrystalReport
End
Else Begin
Send DisplayDialog of oCheckForFlex2Crystal
End
End_Procedure
Embedding a Custom ActiveX Viewer¶
You can embed a preview object into a ReportView. This is an alternative to the popup preview, which is the default method for viewing reports on screen. Simply drag the preview object onto the report and add the Send AssignPreviewObject statement to the OnDisplayReport procedure as shown below.
Procedure OnDisplayReport Handle hoReport
// Called when report is sent to previewer
Forward Send OnDisplayReport hoReport
// This assigns the previewobject so we can use our custom previewer
Send AssignPreviewObject of hoReport oReportPreviewCR
End_Procedure // OnDisplayReport
Using the CDO Interface¶
The Crystal Data Object (CDO) is an ActiveX data source that allows for on-the-fly generated data sets to be used inside a report. The CDO is a way of creating an in-memory array of information and then accessing this information in your report as if it was a table.
Note
Support for CDOs is provided by Flex2Crystal for backwards compatibility. The use of CDOs is considered a legacy implementation.
The data definitions for the in-memory structure (the field name, field type, field length, and sample data) are stored in a TTX file. The TTX file is created by choosing Field Definitions Only in the Database Expert dialog box.
Create a TTX File¶
Open a new or existing report in the Crystal Report Designer.
Open the Database Expert.
In the Available Data Sources panel, expand Create New Connection and then select Field Definitions Only under More Data Sources.
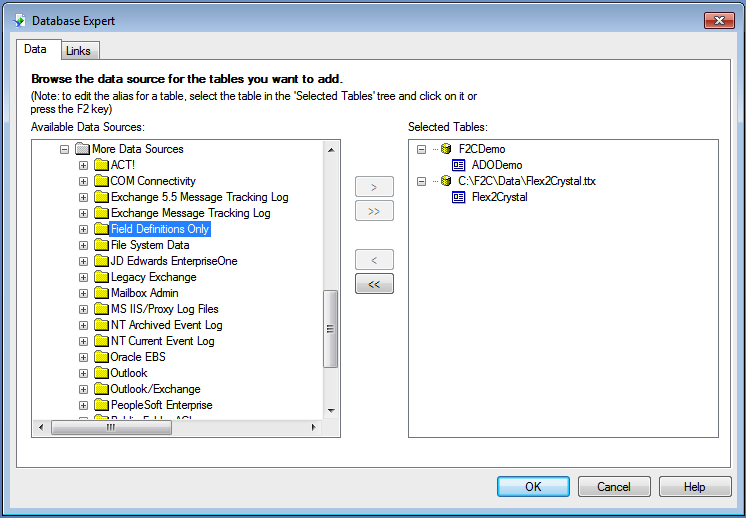
Click OK. The Field Definitions Only dialog box opens.
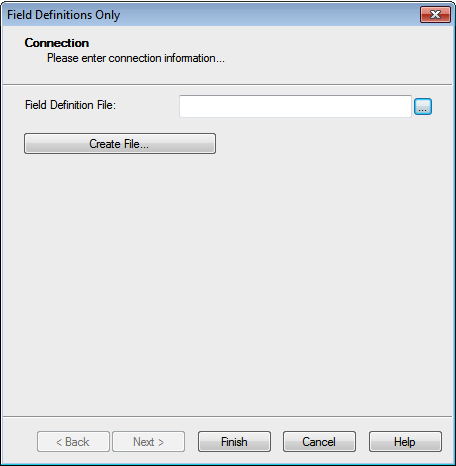
Click the Browse button to open an existing TTX file, or click Create File to create a new TTX file. The Database Definitions Tool dialog box opens.
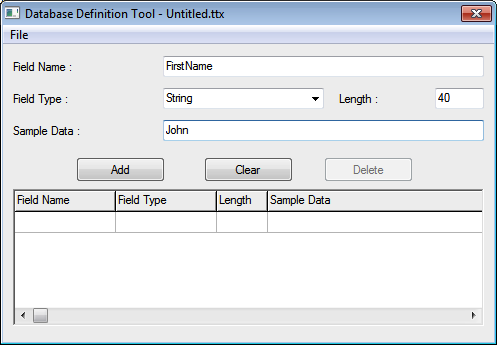
Enter a new field name.
Select the field type using the drop-down list. If the field type is String, enter the field length.
Optionally, enter sample data. The new field definitions appear in the grid at the bottom of the dialog box.
Click Add to add another field. You can also select an existing field and click Update to modify the field properties, or click Delete to delete the field.
When you have finished entering the field definitions, select File > Save As and choose a location for the TTX file. It is recommended that you save the file in your project workspace.
Click Close to close the Database Definitions Tool dialog box.
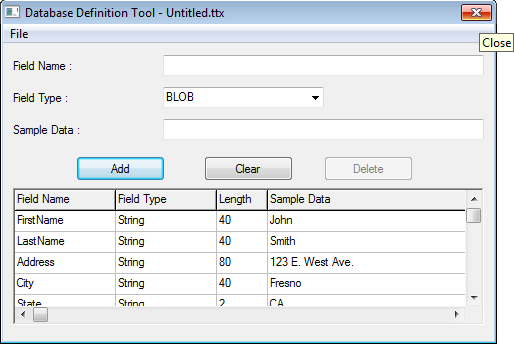
Click Finish in the Field Definitions Only dialog box. The new schema is listed under Available Data Sources.
Using a CDO in Your Report¶
The TTX file defines the layout for the in-memory table. The TTX file does not contain any actual data. The data set is passed to the report using a CDO.
Create the CDO in your report view during the OnInitializeReport method, and then use the AppendCDOData method to map the in-memory array to your report.
The example below creates a CDO based on the data structure stored in Label.ttx.
Function LoadVendors Returns Variant[][5]
Integer iItem iCopies iLabel
Boolean bFound
Variant[][5] vData
Get NumberOfCopies of oCopiesGroup to iCopies
Clear Vendor
Find gt Vendor by 1
Move (Found) to bFound
While bFound
// Must have a City, State, and Zip code to create a label
If ((Vendor.City <> "") and (Vendor.State <> "") and (Vendor.Zip <> "")) Begin
// copy
For iItem From 1 to iCopies
Move (Trim(Vendor.Name)) to vData[iLabel][0]
Move (Trim(Vendor.Address)) to vData[iLabel][1]
Move (Trim(Vendor.City)) to vData[iLabel][2]
Move (Trim(Vendor.State)) to vData[iLabel][3]
Move (Trim(Vendor.Zip)) to vData[iLabel][4]
Increment iLabel
Loop
// Add state to list
Send AddState Vendor.State
End
Find gt Vendor by 1
Move (Found) to bFound
End
Function_Return vData
End_Function // LoadVendors
Procedure OnInitializeReport Handle hoReport
Handle hoLabels
Variant[][5] vData
Get CreateCDO of hoReport "Label.ttx" to hoLabels
If (hoLabels) Begin
Get LoadVendors to vData
Send AppendCDOData of hoReport hoLabels vData
End
End_Procedure // OnInitializeReport
Note
A CDO example is provided in the ReportPreviewCR.rv installed with Flex2Crystal.sws.
Using the ADO.NET Interface¶
ADO.NET (ActiveX Data Objects for .NET) is a set of software components that programmers can use to access and modify data stored in relational databases and non-relational database sources including web pages, spreadsheets, and other types of documents. ADO.NET is a replacement for CDO. ADO.Net uses XML Schema Definitions (XSDs) instead of TTX files to describe the table that is created in memory in DataFlex.
There are two key components to ADO.NET 1) the DataSet and 2) the Data Provider.
Create the DataSet Schema¶
To use the ADO.NET interface you first define the DataSet schema, a template of the data structure in XML format. The XML Schema Definition (XSD) can be defined using the Mertech XSD Generator. The XSD contains only the data description, and not the actual data.
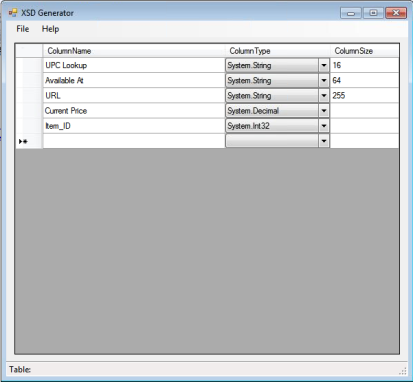
Configure the Connection to the Data Provider¶
Open a new or existing report in the Crystal Report Designer.
Open the Database Expert.
In the Available Data Sources panel, select Create New Connection > ADO.NET (XML) > Make New Connection.
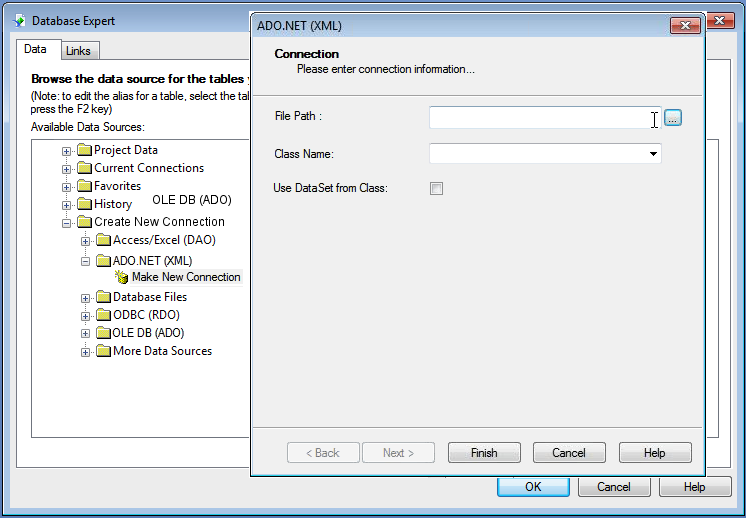
Click the Browse button next to File Path in the displayed Connection dialog box.
Use the drop-down arrow next to Look in to browse for your newly created XSD file (UPC.xsd in this example).
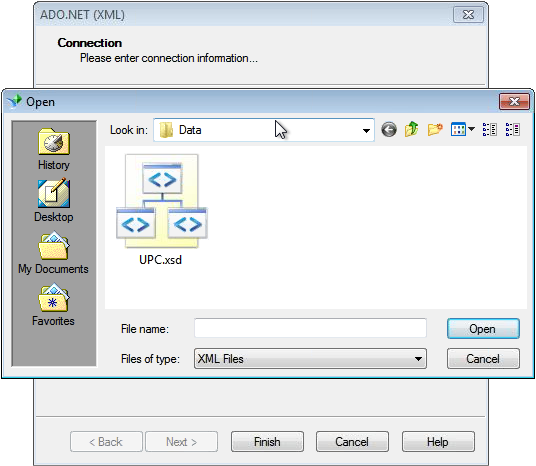
Select the file then click Finish.
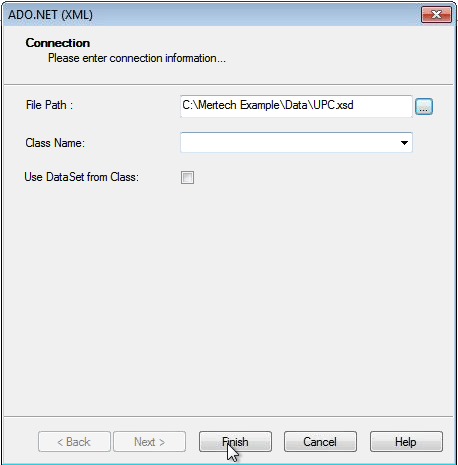
Next, add the data provider (in the example below we are using a LocalDB table) and newly created XSD table (UPC.xsd in the example). Select each table in the Available Data Sources panel then click the right arrow to move the table to the Selected Tables panel.
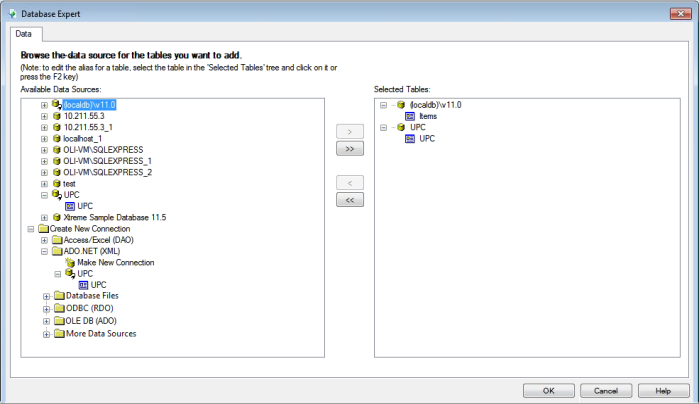
Select the Links tab in the Database Expert dialog box, then select a field in the data table and a corresponding field in the XSD table to link the tables.
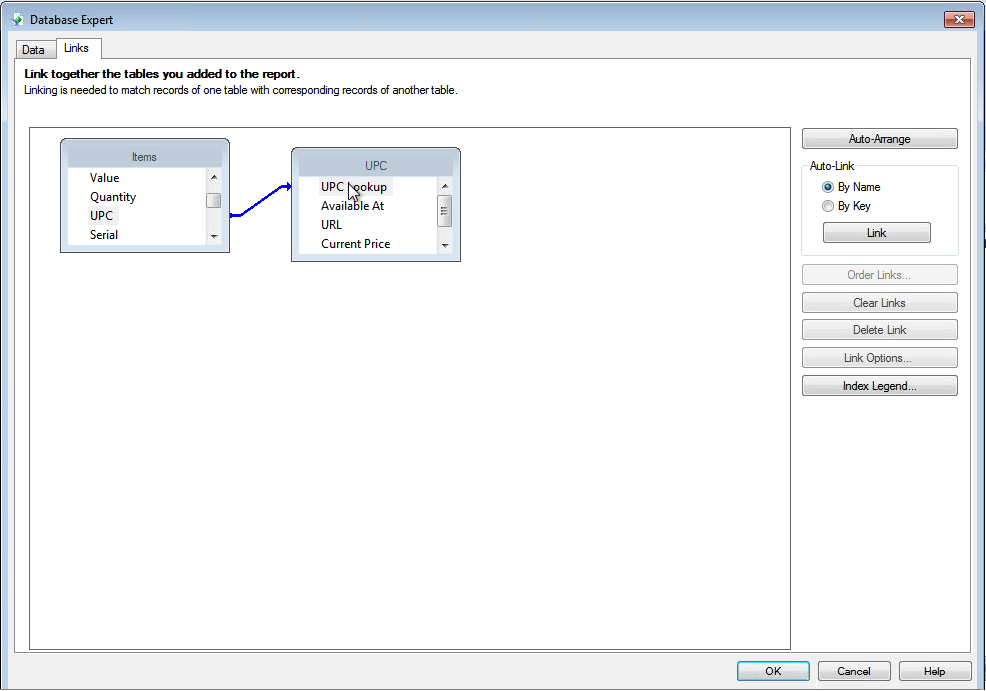
Using ADO.NET in Your Report¶
Modify your report view to use this data source. This requires three steps.
Add the new fields to the report.
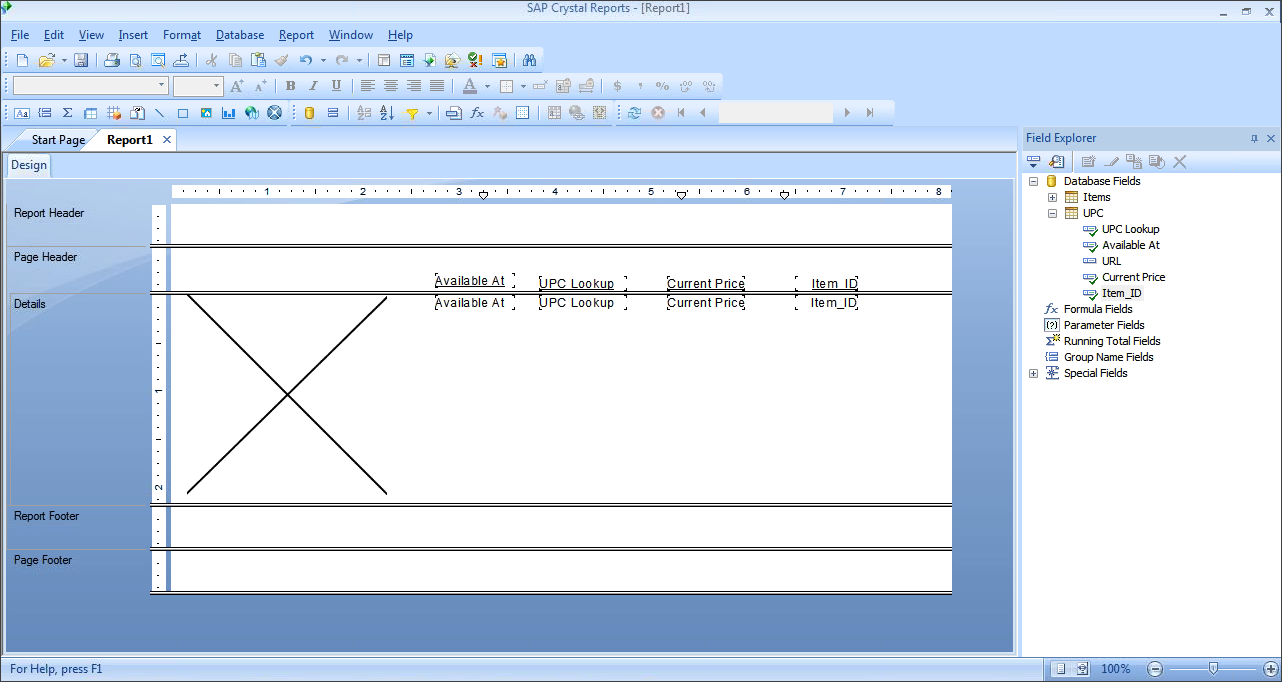
Assign the data source in your report view during the OnInitializeReport method by adding Send AssignADODataSources of hoReport.
Procedure OnInitializeReport Handle hoReport
Send AssignADODataSources of hoReport
End_Procedure
Create a procedure to add the table rows. The LoadRowData example below adds rows to a table.
Procedure LoadRowData Handle hoDataTable String sTable
String upc sRetVal
Integer id
Variant vRows vRow
Variant[5] vData
Handle hoRows hoRow
tProductDetails prod
Get ComRows of hoDataTable to vRows
Get Create U_cComF2CDataRowCollection to hoRows
Set pvComObject of hoRows to vRows
Get Create U_cComF2cDataRow to hoRow
If (sTable = "UPC") Begin
SQL_SET_STMT to "SELECT UPC, ITEM_ID FROM ITEMS WHERE UPC <> ' ' "
SQL_PREPARE_STMT CURSOR_TYPE TYPE_CLIENT
SQL_EXECUTE_STMT
Repeat
SQL_FETCH_NEXT_ROW INTO upc id
If (Found) Begin
Get ProductDetails upc to prod
Move prod.UPC to vData[0]
Move prod.available at to vData[1]
Move prod.imageurl to vData[2]
Move prod.price to vData[3]
Move id.UPC to vData[4]
Get ComNewRow of hoDataTable to vRow
Set pvComObject of hoRow to vRow
Set ComItemArray of hoRow to vData
Send ComAdd of hoRows vRow
End
Until (FindErr)
End
End_Procedure
Note
The above example uses a LocalDB as the data source. The complete report, ReplacePricing.rv, is available in the Mertech Example Application.sws workspace. An example using an XML file as the data source is provided in the ReportPreviewCR.rv, also installed with Flex2Crystal.sws. Use of the XML file requires casting each element to the proper type for that column since the data is in string format.